People feel the game will loose if too much automation
creeps in. Still there are times we wish boats had a
little intelligence of their own.
Going hours with the wrong sail?
Or getting stuck against the wind during the night?
Can a Captn get a decent sleep???
Enter Boat Script. BS is a script system designed to facilitate
monitoring and controlling boats in vrtool.
It uses JavaScript, a powerful and popular script language.
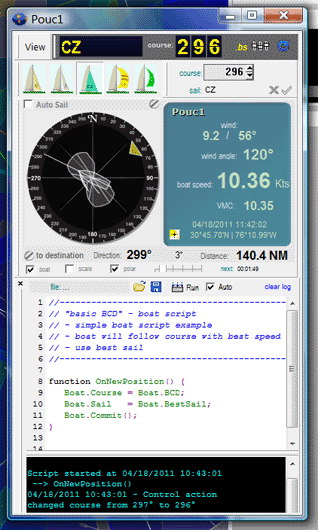
Only vrtool's own sandbox boats can be controlled (course/sail).
Other boats can be monitored. Scripts always run in the client machine.
Running untrusted scripts (like web browsers do) is tricky.
The following illustrates a simple Boat Script:
- Code: Select all
function OnNewPosition() {
Boat.Course = Boat.BCD;
Boat.Sail = Boat.BestSail;
Boat.Commit();
}
Event OnNewPosition() is fired when a new point is
added to boat track (a 10 min tick)
What this script does?
1- Sets boat course to the best course to destination (best velocity made good)
2- Selects the best sail for that course
3- Uses Commit() to send the control action to the server (if course and/or sail changed)
Only 5 lines, yet pretty efficient. It will even enter zig-zag mode as needed (see image below)
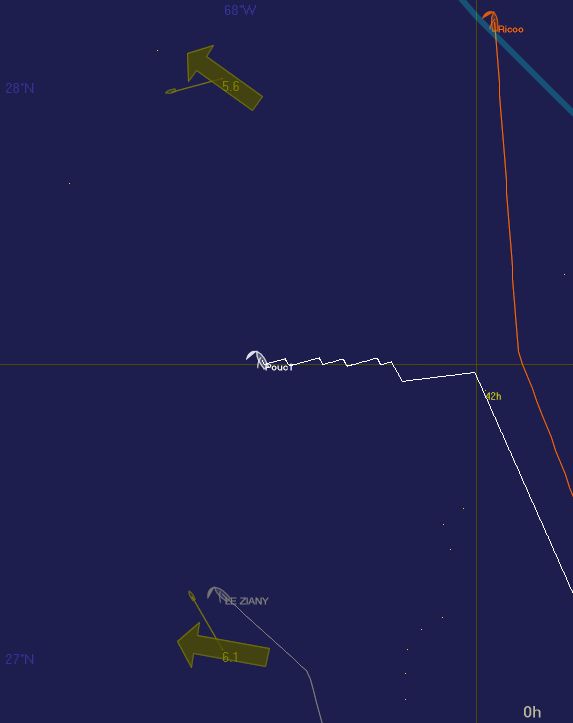
The full BoatScript interface is below:
Control Parameters (read/write):
- Code: Select all
Boat.Course; int
Boat.Sail; int - Numeric sail ID
Boat.Commit(); Sends changed control parameters to server (if course or sail changed)
Boat data properties (read only):
- Code: Select all
Boat.Boatspeed; double in knts
Boat.Latitude; double
Boat.Longitude; double
Boat.TWS; double Wind speed in knts
Boat.TWD; int Wind direction
Boat.TWA; int Wind angle
Boat.Classif; int Classification
Boat.DestDirection; int Direction to destination
Boat.DestDistance; double Distance to destination in NM
Boat.BCD; int Best course to destination
Boat.VMC; double Velocity made good to destinatio
Boat.BestSail; int Best sail for the course
Boat.PosTime; datetime Position time
Boat.Boatname; String Boat name
Boat.Sailname; String Sail name
Query other boats:
- Code: Select all
Boat.BoatDistance(Boatname); double Distance to another boat in NM (returns 0 if boat not found)
Boat.BoatDirection(Boatname); int Direction to another boat (returns -1 if boat not found)
Boat.PointDistance(lat,lon); double Distance to a point in NM
Boat.PointDirection(lat,lon); int Direction to a point
Boat.GetBoat(Boatname); returns interface to another Boat (null if boat not found)
You can use other boat data in your script, but cannot commit changes
to control parameters in other boats (of course).
Script logging:
- Code: Select all
Boat.ShowData(); Show boat data in script log
Boat.ShowMessage(Msg); Show message in script log
Boat.ClearMessages(); Clear script log
Events:
- Code: Select all
function OnNewPosition() {
//fired every time a new point is received
}
// the texts after two slashes are comments (not necessary to run the script)
Notes: * All distances and directions calculated with great circle formulas
* South latitudes and East longitudes are negative
Script examples:
corkscrew route:
- Code: Select all
// "corkscrew route" - boat script
// - increasing course regularly ( 2 degrees/10 min tick )
// - always use best sail (screw the crew)
// - skip pointing upwind
function OnNewPosition() {
Boat.Course = Boat.Course+2; // increase course each tick
if (Boat.TWA<45) { // skip upwind (wind angle<45)
Boat.Course = Boat.Course+90;
Boat.ShowMessage("Tack!");
}
Boat.Sail = Boat.BestSail; //change sail if needed
//print information
Boat.ShowData();
Boat.ShowMessage("Hi Captn. Doing good at "+Boat.Boatspeed+" knts");
Boat.Commit(); // sends control params, if changed
}
Follow a boat (either local or VR)
- Code: Select all
// "bs_followTitanic.js" - Follows a boat named "Titanic" with best sail
function OnNewPosition() {
var c = Boat.BoatDirection("Titanic"); // BoatDirection() returns -1 if boat not found
if (c>=0) {
Boat.Course = c;
Boat.Sail = Boat.BestSail;
Boat.Commit();
}
}
Print VMC table:
- Code: Select all
// "print VMC table" - boat script
// - this example prints a table with course;vmc columns
// - example of how to use the script log
// - does not affect boat control ( since there is no commit() command )
function OnNewPosition() {
var bestSpeed=0;
var bestCourse=0;
var vmc;
for (i=0; i<360; i++) {
Boat.Course = i;
vmc = Boat.VMC;
if (vmc>bestSpeed) {
bestSpeed=vmc;
bestCourse=i;
}
Boat.ShowMessage(i+";"+vmc);
}
Boat.ShowMessage("best course="+bestCourse+"/best vmc="+bestSpeed);
}
Installation
BoatScript is available in version 1.97c (zip)
link: http://www.tecepe.com.br/nav/vrtool/vrtool.zip
md5: 213583286F3351A5D95013493E4A2F13
Some sample scripts included (search for bs_*.js)
Users with versions older than 1.97b should use the full installer first,
then unzip the latest 1.97c
Usage
Use BoatScript panel in Remote Boat control window, as illustrated below.
Important: Remember to save the script.
I use files named bs_scriptname.js
Scripts are saved as external .js files, not embedded in the desktop.
So any change must be saved to the script file to remain persistent.
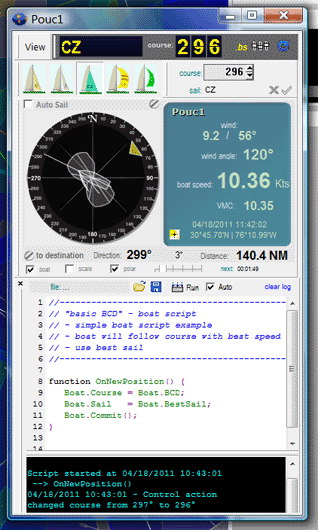